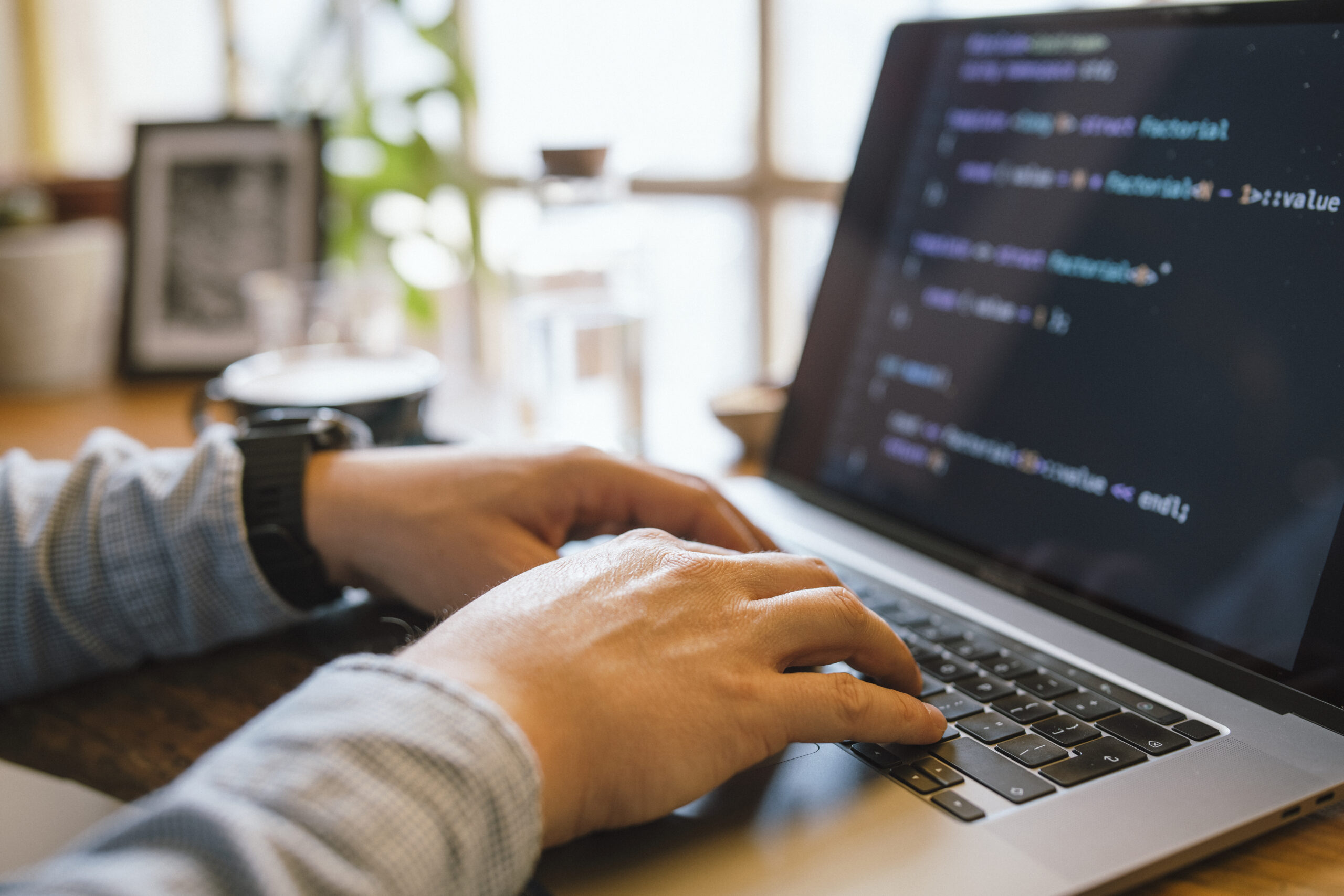
Debugging is Just about the most critical — yet usually neglected — competencies in a developer’s toolkit. It isn't nearly correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Consider methodically to unravel complications competently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and substantially increase your productiveness. Listed below are various tactics that can help developers degree up their debugging game by me, Gustavo Woltmann.
Learn Your Resources
Among the fastest strategies builders can elevate their debugging expertise is by mastering the resources they use every single day. Although writing code is one Element of progress, being aware of tips on how to communicate with it successfully all through execution is Similarly essential. Modern progress environments occur Outfitted with powerful debugging capabilities — but many developers only scratch the surface area of what these instruments can perform.
Choose, one example is, an Integrated Progress Environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These equipment let you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in many cases modify code within the fly. When made use of accurately, they Enable you to observe particularly how your code behaves in the course of execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-close developers. They assist you to inspect the DOM, check community requests, look at real-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn disheartening UI concerns into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these applications could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with version Handle programs like Git to be familiar with code history, discover the exact second bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment suggests likely further than default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment to make sure that when problems come up, you’re not misplaced at nighttime. The higher you understand your equipment, the more time you may shell out fixing the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Just before jumping into the code or earning guesses, builders need to have to create a consistent surroundings or scenario where by the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of prospect, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating just as much context as you can. Inquire thoughts like: What steps led to The difficulty? Which surroundings was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it gets to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected plenty of details, seek to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating equivalent user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the sting cases or condition transitions associated. These tests not merely assistance expose the trouble and also avoid regressions Down the road.
Occasionally, The problem can be atmosphere-distinct — it'd come about only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the problem isn’t just a stage — it’s a frame of mind. It requires persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to repairing it. That has a reproducible state of affairs, you can use your debugging tools much more successfully, check opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary complaint right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Error messages tend to be the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, builders really should understand to treat error messages as immediate communications through the program. They generally let you know just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start by examining the concept cautiously As well as in entire. Numerous builders, particularly when beneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs might lie the legitimate root lead to. Don’t just copy and paste error messages into search engines like yahoo — read and fully grasp them initial.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also helpful to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to recognize these can substantially increase your debugging method.
Some glitches are imprecise or generic, and in People conditions, it’s essential to look at the context by which the error transpired. Look at associated log entries, input values, and up to date variations while in the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial challenges and supply hints about possible bugs.
Eventually, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint issues quicker, minimize debugging time, and become a far more efficient and confident developer.
Use Logging Wisely
Logging is Just about the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A great logging approach begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like effective start-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine troubles, and FATAL in the event the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Far too much logging can obscure significant messages and slow down your system. Deal with vital gatherings, state changes, enter/output values, and critical conclusion factors in your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. By using a perfectly-believed-out logging tactic, you can decrease the time it will require to identify problems, achieve deeper visibility into your programs, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a specialized undertaking—it's a kind of investigation. To proficiently identify and repair bugs, developers have to solution the process like a detective solving a secret. This mindset assists break down sophisticated troubles into workable pieces and abide by clues logically to uncover the root trigger.
Start out by accumulating proof. Think about the symptoms of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, accumulate just as much suitable information and facts as you could without the need of leaping to conclusions. Use logs, take a look at conditions, and person stories to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Question on your own: What might be leading to this conduct? Have any modifications not too long ago been created to the codebase? Has this difficulty happened ahead of less than very similar conditions? The objective is to narrow down possibilities and determine potential culprits.
Then, take a look at your theories systematically. Try and recreate the issue in a managed ecosystem. In case you suspect a certain perform or element, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code queries and let the final results lead you nearer to the reality.
Pay out shut consideration to little aspects. Bugs typically hide from the least envisioned spots—just like a missing semicolon, an off-by-one particular error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly knowing it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming issues and support others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, strategy challenges methodically, and become simpler at uncovering hidden difficulties in complex techniques.
Publish Checks
Writing tests is one of the simplest ways to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early and also function a security Web that gives you self-confidence when producing adjustments to the codebase. A very well-analyzed software is simpler to debug as it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with unit tests, which focus on individual capabilities or modules. These compact, isolated checks can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a examination fails, you quickly know in which to appear, considerably reducing time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration tests and conclusion-to-conclude exams into your workflow. These help make sure a variety of elements of your software get the job done collectively smoothly. They’re specially beneficial for catching bugs that arise in complicated systems with a number of components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing assessments also forces you to definitely Consider critically about your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to deal with repairing the bug and look at your exam pass when The problem is solved. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from a discouraging guessing activity into a structured and predictable method—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to become immersed in the situation—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and often see the issue from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different endeavor for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mindset. You would possibly abruptly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. get more info Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it basically results in speedier and more effective debugging In the long term.
In short, having breaks isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is part of fixing it.
Study From Each Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can teach you one thing precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring yourself a couple of important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots inside your workflow or comprehending and assist you to Develop more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In group environments, sharing Everything you've learned from the bug using your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
Far more importantly, viewing bugs as lessons shifts your way of thinking from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial parts of your growth journey. In the end, a lot of the greatest builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques requires time, follow, and tolerance — however the payoff is big. It makes you a more productive, self-assured, and capable developer. The next time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.